First Steps with the STM32F4 in Linux (Part 1)
June 1, 2012
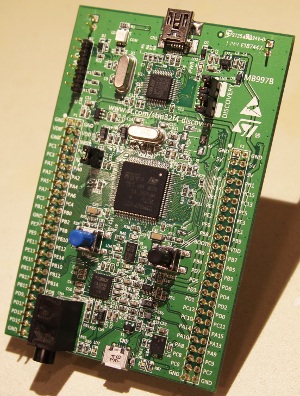
The Arduino is easy and fun to use but eventually a project will come along that needs more power. There are plenty of Arduino-compatible boards that use a more powerful PIC or ARM microcontroller and preserve the ease of use. Those are probably great options, but I wanted to step outside of that comfort-zone and learn the ARM architecture from the ground up. It's a steep learning curve, but very fun and rewarding if you aren't in a rush.
The STM32F4DISCOVERY development board turned out to be a great option:
ARM Cortex-M4F with FPU (32-bit, adjustable clock up to 168MHz)
1MB Flash, 192+4KB SRAM
12-bit ADCs, 12-bit DACs
17 timers
5V tolerant IO
I2C, I2S, USART, UART, SPI, USB and lots more.
MEMS digital accelerometer
MEMS digital microphone
Audio DAC with class D speaker driver
ST-LINK V2 built-in
Four LEDS and a pushbutton
At $17 it's hard to pass up. Unfortunately there are two noteworthy issues. STM does not support any open-source or free development environments. The four that are supported (TrueSTUDIO, TASKING, MDK-ARM and EWARM) aren't cheap however there are limited demo versions available for free if you use Windows. The other issue is a lack of documentation for people still learning how to use an MCU. I don't blame ST for this since they don't market it for beginners, but it is an issue for people who want to transition out of easy-to-use MCU environments like the Arduino or BS2.
Let's install the toolchain, ST-link utility, build an example project and go over some of the documentation and firmware packages. There are several options for the toolchain and the two most popular seem to be Summon ARM Toolchain and GCC ARM Embedded. SAT downloads the source packages for all of the tools and automates the compilation process. GCC ARM Embedded comes as a precompiled archive that you simply extract and add to your $PATH. I decided to use GCC Arm Embedded but directions for both are listed below. Only install one toolchain!
Install the Toolchain - Summon ARM Toolchain
Install some dependencies from the Ubuntu repositories, download SAT, compile it and add the SAT path to your $PATH environment variable:
$ sudo apt-get install flex bison libgmp3-dev libmpfr-dev libncurses5-dev libmpc-dev autoconf \
texinfo build-essential libftdi-dev libsgutils2-dev zlib1g-dev libusb-1.0-0-dev git
$ mkdir ~/stm32f4
$ cd ~/stm32f4
$ git clone https://github.com/esden/summon-arm-toolchain.git
$ cd summon-arm-toolchain
$ sudo ./summon-arm-toolchain
$ export PATH=~/sat/bin:$PATH
$ echo export PATH=~/sat/bin:\$PATH >> ~/.profile
Install the Toolchain - GCC ARM Embedded
Download the archive, extract it and add the GCC ARM Embedded path to your $PATH environment variable:
$ mkdir ~/stm32f4
$ cd ~/stm32f4
$ wget https://launchpad.net/gcc-arm-embedded/4.6/4.6-2012-q1-update/+download/\
gcc-arm-none-eabi-4_6-2012q1-20120316.tar.bz2
$ tar -xjvf gcc-arm-none-eabi-4_6-2012q1-20120316.tar.bz2
$ export PATH=~/stm32f4/gcc-arm-none-eabi-4_6-2012q1/bin:$PATH
$ echo export PATH=~/stm32f4/gcc-arm-none-eabi-4_6-2012q1/bin:\$PATH >> ~/.profile
The latest version will probably be different. Download the latest version from https://launchpad.net/gcc-arm-embedded and modify the extract/$PATH commands listed above accordingly.
Install ST-link
Install libusb-dev and git from the Ubuntu repositories, download stlink, compile it and allow device access for regular users:
$ sudo apt-get install libusb-1.0-0-dev git
$ cd ~/stm32f4
$ git clone https://github.com/texane/stlink.git
$ cd stlink
$ ./autogen.sh
$ ./configure
$ make
$ sudo make install
$ sudo cp 49-stlinkv1.rules /etc/udev/rules.d/
$ sudo cp 49-stlinkv2.rules /etc/udev/rules.d/
$ sudo udevadm control --reload-rules
Build an Example Project
STM has a firmware library (more on that later) but it is not setup for use with Linux or any of the open-source IDEs. The stlink package includes a copy of the firmware with Makefiles and linker scripts, and Karl Palsson has collected/modified/written a nice collection that builds on top of that. It's a work-in-progress and his collection is available on GitHub. He also has a blog that covers the STM32 series and lots of other stuff. Check it out: http://false.ekta.is/category/stm32/
Download his collection, build the STM Standard Peripheral Drivers, build the IO_Toggle project, and upload the resulting firmware to the board:
$ cd ~/stm32f4
$ git clone git://github.com/karlp/kkstm32_base
$ cd kkstm32_base/example/stm32f4/STM32F4xx_StdPeriph_Driver/build/
$ make
$ cd ../../Projects/IO_Toggle/
$ make
$ st-flash write IO_Toggle.bin 0x08000000
If everything worked correctly the last line printed to the terminal will be “...Flash written and verified! jolly good!” The example project justs blinks the four LEDs located around the accelerometer in a circular fashion.
Documentation and Official Firmware Packages
There are three main sources of documentation: STM's page for the microcontroller, STM's page for the development board, and ARM's page for the Cortex-M4 (STM's chip is built on ARM's platform.) Among the dozens of files, I found these to be the most helpful for getting started:
Microcontroller: http://www.st.com/internet/mcu/subclass/1521.jsp (click on the Resources tab)
RM0090 Reference Manual for the STM32F4 series. It covers everything. Start here:
Memory map on p.50 (Section 2.3)
Reset and clock control register map on p.134 (Section 5.3.24)
GPIO register map on p.153 (Section 6.4.11)
PM0214 Programming Manual for the STM32F4 series
Covers assembly language and how the processor works.
DM00037051 Datasheet for the STM32F407xx and STM32F405xx series
Specifications and technical information.
stm32f4_dsp_stdperiph_lib.zip
General firmware package with project files for Windows IDEs.
Development Board: http://www.st.com/internet/evalboard/product/252419.jsp (click on the Design Support tab)
UM1472 User Manual for the STM32F4DISCOVERY board
General introduction, schematic and board details.
Extension Connectors table on p.20 (Section 4.12) covers what each pin can do.
stm32f4discovery_fw.zip
Firmware collection that highlights the development board peripherals.
ARM: http://www.arm.com/products/processors/cortex-m/cortex-m4-processor.php
DDI0439C Cortex-M4 Technical Reference Manual
Everything builds upon this specification.